Built-in functions
Blockpad has hundreds of built-in functions, ranging from basic functions like Sqrt() to those made specifically for engineering, like VlookupInterpolate().
This deep dive page is not an exhaustive list of all Blockpad functions, but it gives an overview of commonly used functions and an idea of how to use them.
The majority of Blockpad functions are made to work like conventional spreadsheet functions, e.g. If(), Vlookup(), AverageIf(), or Concat(). For these functions, you can typically reference the large amount of online content covering how best to work with them, in addition to Blockpad documentation.
Blockpad also makes it easy to define your own custom functions. Learn more in the calculations section of the deep dive.
Functions as library items
Blockpad functions are stored in different libraries that are included in the program by default. You can view the libraries, and see all of the functions they contain, on the Blockpad library page under the Built-in section.
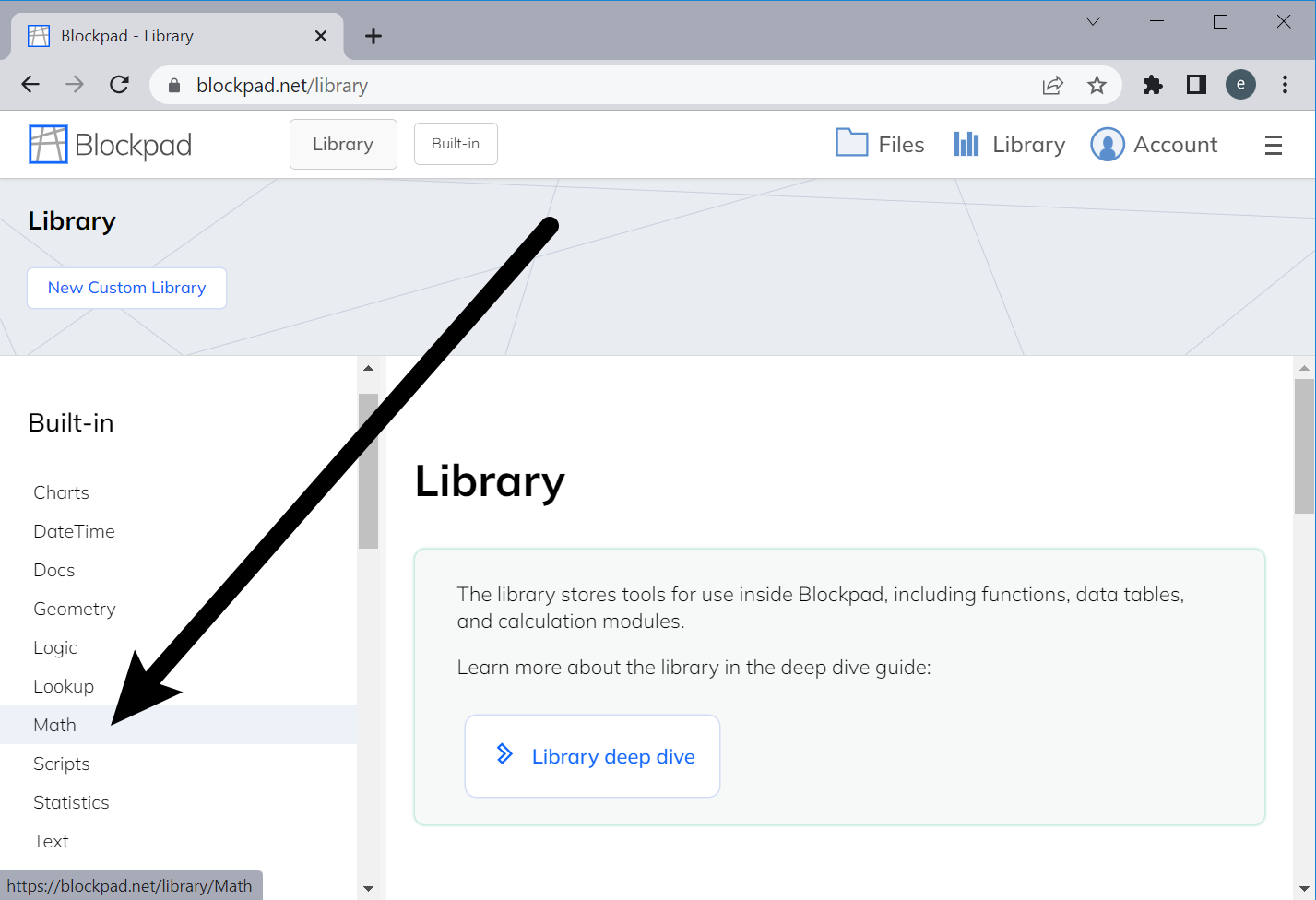
Further documentation on each function can be found on it's respective library page. The link provided in the tables below will take you to the page for that function.
Functions and units
Blockpad functions are built to work with units intelligence. In practice, this means different things for different functions.
For example, Sum() or Average() will automatically convert all inputs to the same output unit, but they need to all be compatible with each other (e.g. ft and m, or psi and MPa).
Other functions will only take specific units as an input. For example, the inputs for Sin() must have angular-compatible units.
The way functions work with units should be intuitive, but you can check the function documentation or the units page for information.
Math
Basic math functions
Blockpad includes standard, basic math functions like Sqrt(), Log(), and Abs(). Some of these are listed below.
Sqrt() | Returns the square root of a number. |
Log() | Returns the log of a number, given a base. |
Ln() | Returns the natural log of a number. |
Abs() | Returns the absolute value of a number. |
Math Values
Blockpad includes built-in values. Unlike conventional spreadsheets, they are stored as values, not functions, so there's no need to follow them with parentheses.
pi or π | The value for pi, i.e. 3.14159... |
e | The value for Euler's number, i.e. 2.71828... |
i or j | The imaginary number, i.e. the square root of negative one. |
Trigonometry
Blockpad includes all trigonometric and and hyperbolic functions.
The inputs for trig functions are expected to have an angular unit (rad or deg). If no unit is provided, the input will be interpreted as radians.
The inputs for inverse trig functions are expected to be unitless and in the range acceptable for that function (e.g. between -1 and 1 for acos()). The outputs for inverse trig functions are given as radians, but can be converted to degrees using the to keyword.
Trigonometric functions also work with complex numbers.
Sin() | Returns the sine of an angle. |
Cos() | Returns the cosine of an angle. |
Tan() | Returns the tangent of an angle. |
Asin() | Returns the arcsine of a number as an angle between -pi/2 and pi/2. |
Acos() | Returns the arccosine of a number as an angle between 0 and pi. |
Atan() | Returns the arctangent of a number as an angle between -pi/2 and pi/2. |
Atan2() | Returns the arctangent of given (x, y) coordinates as an angle between -pi and pi, not including -pi. |
Csc() | Returns the cosecant of an angle. |
Sec() | Returns the secant of an angle. |
Cot() | Returns the cotangent of an angle. |
Sinh() | Returns the hyperbolic sine of an angle. |
Cosh() | Returns the hyperbolic cosine of an angle. |
Tanh() | Returns the hyperbolic tangent of an angle. |
Asinh() | Returns the hyperbolic arcsine of a number as an angle between -pi/2 and pi/2. |
Acosh() | Returns the hyperbolic arccosine of a number as an angle between 0 and pi. |
Atanh() | Returns the hyperbolic arctangent of a number as an angle between -pi/2 and pi/2. |
Functions for units
Compatible() | Tests if two numbers have compatible units (e.g. both are lengths) and returns true or talse. Compatible(1ft, 3m) = true |
Magnitude() | Returns the unitless magnitude of a number with units. ***The preferred method for "stripping" units is found on the units page |
Unit() | Returns the units stored with a number. |
UnitConvert() | Converts a number to different units. (e.g. UnitConvert(5 m, 1 in) )The to keyword is typically preferable (e.g. 5 m to in ). |
Rounding functions
Round() | Returns a value rounded to a specified number of digits. |
RoundDown() | Returns a value rounded down to a specified number of digits. |
RoundUp() | Returns a value rounded up to a specified number of digits. |
RoundSigFigs() | Returns a value rounded to the specified number of significant figures. |
Calculus
Derivative() | Returns the derivative of a function. Derivative(x=>x^2+x) = 2*x + 1
|
Integral() | Returns the definite integral of a function, given start and end points. Integral(x=>2*x+3, 1, 4) = 24
|
The main input for Derivative() and Integral() is a custom function. This function can either be a named custom function, or an inline function directly in the input.
The output for Derivate() is a function that can be used in formulas. Just reference the result, and use it like a normal function.
Also note that the units of the bounds for the Integral() function have an impact on the result, but you must make sure the units of the function all work out.
Interpolation
Interpolate() | Classic interpolation - returns a y value given an x value and pairs of x and y (x1, x2, y1, y2). Interpolate(2.5, 1, 5, 10, 50) = 25
|
VlookupInterpolate() | Returns an interpolated value, given a lookup value, an array or range, and a column index. |
HlookupInterpolate() | Returns an interpolated value, given a lookup value, an array or range, and a row index. |
Interpolate2D() | Returns a value given a row lookup value, a column lookup value, and an array or range. |
Note: VlookupInterpolate() and HlookupInterpolate() are stored in the Lookup library, and not the Math library.
VlookupInterpolate() Example
Interpolate2D() Example
Numerical solving
In addition to solver sections, Blockpad has a GoalSeek() function for finding numerical solutions to single variable functions.
GoalSeek() | Returns the value that when plugged into the given function, yields the desired solution.GoalSeek(x=>x^2+x, 6) = 2
|
The first input for GoalSeek() is a single variable function, which can be either a named custom function or an inline function. The second input is the desired result for that function, and the output is a value of the function variable that would yield that result.
Because GoalSeek() is a numerical solver, it does not provide all solutions to an equation, only one.
Summation
In Blockpad, summation is available as a function, and when it's entered in a [dynamic equation], it's displayed with typical math notation using the sigma symbol.
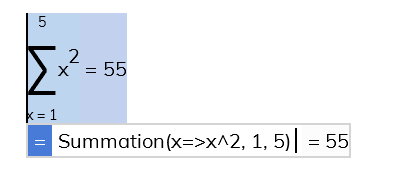
The first input to Summation() is a function, typically an [in-line function], but named functions work too. The second and third inputs are the start and end, respectively, of the series.
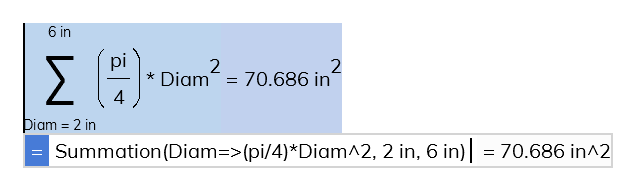
You can create double summations by layering two summation functions, as shown in the example below.
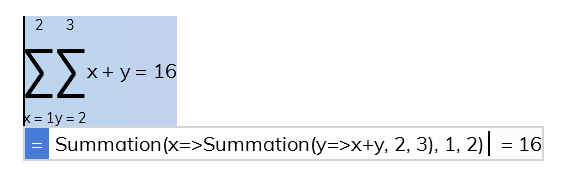
Logic
For the most part, Blockpad logic functions work like conventional spreadsheet logic functions.
You can read more about boolean operations in the Logic and boolean values section of the deep dive.
Basic logic functions
Not() | Returns true if given false, and false if given true.Not(0 > 100) = true Not("A"=="A") = false |
And() | Returns true if all inputs are true, and false if any are false.And(100 > 0, 0 > -100) = true And("A"=="A", "A"=="B") = false |
Or() | Returns true if any inputs are true, and false if none are true.Or(100 < 0, 0 < -100) = false Or("A"=="A", "A"=="B") = true |
Xor() | Returns true if there are an odd number of true inputs.Xor(100 > 0, 0 > -100) = false Xor("A"=="A", "A"=="B") = true |
If()
The Blockpad If() function
works just like the If() function in conventional spreadsheets.
Given a boolean value, it returns one thing if true, and another thing if false.
E.g. If(5>0, "positive", "negative") = "positive"
When used in dynamic equations, the If() function is displayed as a logic table.
When chained If() functions are used, the logic table continues.
E.g. If(condition1, result1, If(condition2, result2, resultIfConditionsNotMet))
These logic tables can be read as "Return A, if X condition is met; Return B if Y condition is met; etc". The example below could be read as "k_c = 1 if loading type is bending; k_c = 0.85 if loading type is axial; etc."
The actual formulas for chained If() functions can get very long. To break them up and make them easier to read, you can use shift+enter in the formula to create a new line.
It can also be helpful to use the visual editor.
Text
Blockpad text functions are mostly modeled after conventional spreadsheet text functions, with some different functions available.
Find more on text values in the text values section of the deep dive.
Basic text functions
Concat() | Returns the given text values combined as one text value.Concat("hello", " ", "there") = "hello there" |
Left() | Returns a specified number of characters from the left of a given text value.Left(5, "hello there") = "hello" |
Right() | Returns a specified number of characters from the right of a given text value.Right(5, "hello there") = "there" |
Mid() | Returns a specified number of characters from the middle of a text value, given a starting point.Mid("hello there", 3, 8) = "llo ther" |
Len() | Returns the number of characters in a given text value.Len("hello there") = 11 |
Text() | Returns the given value as text.Text(102.1 lb) = "102.1 lb" |
Value() | Returns a parsed number value from a given text value.Value("102.1 lb") = 102.1 lb |
Trim() | Returns all spaces from a text value except single spaces between characters.Trim(" hello there ") = "hello there" |
Upper() | Returns a given text value in all upper case letters.Upper("Hello there") = "HELLO THERE" |
Lower() | Returns a given text value in all lower case letters.Lower("Hello there") = "hello there" |
More text functions
Replace() | Returns a text value with a given sub-string replaced with a different sub-string.Replace("hello there", " there", ", good morning") = "hello, good morning" |
Find() | Returns the starting location of a text sub-string in a given text value. Case sensitive.Find("hello", "Hello hello there") = 7 |
Search() | Returns the starting location of a text sub-string in a given text value. Not case sensitive.Search("hello", "Hello hello there") = 1 |
TextJoin() | Returns a text value created from a given array, with a delimiter between each item.TextJoin("_", ["Hello", "hello", "there"]) = "Hello_hello_there" |
TextJoin2() | Returns a text value created from a given array, with a different delimiter between columns and rows.TextJoin2("; ", "=", ["A", "B"; "C", "D"]) = "A=B; C=D" |
TextSplit() | Returns a one dimensional array created from splitting a text value at the given delimiter.TextSplit(" ", "Hello hello there") = ["Hello", "hello", "there"] |
TextSplit2() | Returns two dimensional array created from splitting a text value into columns and rows based on the given delimiters.TextSplit2("; ", "=", "A=B; C=D") = ["A", "B"; "C", "D"] |
Unicode() | Returns the unicode value of the first character in a given text value.Unicode("hello there") = 104 |
Char() | Returns the character of a unicode value (given as a unitless number).Char(104) = "h" |
Table of Contents
-
- Start a new document
- Dynamic equations
- Unit tracking
- Value names
- Reference unnamed values
- Text values
- Other value types
- Referencing a value
- Value name rules
- Names used multiple times
- Renaming and auto-updated references
- Built in values
- Visual editor
- Math layout options
- Show steps and more
- Value formatting
-
- Text values
- Date and time arithmetic
- Logic and Boolean values
- Matrices and arrays
- Enter an array or matrix
- Array names
- Matrix calculations
- Item-by-item calculations
- Arrays - more than just numbers
- Array formulas in spreadsheets
- Array parentheses lookup
- Assign individual value names
- Advanced array functions
- Complex numbers
- Spreadsheet in a file
- Sort and filter
- Conditional formatting
- Blockpad specific features
- Open a CSV file
- Mini-spreadsheets in a document
- Reports in a file
- Header and footer
- Page layout properties
- Comments
- Document navigation
- Document-wide formatting
- Start a drawing
- Drawing objects
- Lines, points, and shapes
- Text labels
- Linear dimension labels
- Textboxes
- Images
- Selecting multiple objects
- Ordering objects
- Using the point snapping
- Points you can snap to
- Horizontal and vertical from points
- Parallel or perpendicular lines
- Point snap options
- Transformations - resizing and moving
- Format drawings
- Keyboard input and canvas scales
- Notebooks in a file
- Reference values from other top level frames
- Working in a notebook
- Notebook pages
- Top level frames
- Frames in Frames
- Value containers and location
- Frames and sections are containers
- Containers inside containers
- Dot notation to specify a value in a container
- Capture values
- What is a block?
- Block example
- Use the block results
- Block inputs
- View block as table
- Block instances and block definitions
- More examples
- Create a block
- When to make a block
- Make a block with Blockpad calculations
- Update a block definition
- Create a block using scripts
- Block tables
- Examples library
- Use library items in a document
- Use the long form library item name
- Assign a shortcut name to the library
- Include a library in a file
- Use a library number value
- Use a library function
- Use items in a library frame
- Use a library data table as function
- Use library blocks
- Core, built-in, and subscription libraries
- Create personal libraries
- Save items to your library
- Manage your library online
- Library subscriptions
- Console basics
- Read values from the console
- Overwrite values from the console
- Use read and write to manipulate values
- Assign units to numbers in a cell range
- Stemscript basics for scripts
- Macros
- Module functions